diff --git a/.github/scripts/setup_examples_test.bash b/.github/scripts/setup_examples_test.bash
new file mode 100755
index 00000000..4c3080b0
--- /dev/null
+++ b/.github/scripts/setup_examples_test.bash
@@ -0,0 +1,12 @@
+#!/usr/bin/env bash
+
+for f in *; do
+ if [ -d "$f" ]; then
+ # Will not run if no directories are available
+ go mod init
+ go mod download
+ go run .
+ fi
+done
+
+# git update-index --chmod=+x ./.github/scripts/setup_examples_test.bash
\ No newline at end of file
diff --git a/.github/workflows/ci.yml b/.github/workflows/ci.yml
new file mode 100644
index 00000000..5d5c2369
--- /dev/null
+++ b/.github/workflows/ci.yml
@@ -0,0 +1,34 @@
+name: CI
+
+on:
+ push:
+ branches: [ main ]
+ pull_request:
+ branches: [ main ]
+
+jobs:
+
+ test:
+ name: Test
+ runs-on: ubuntu-latest
+
+ strategy:
+ matrix:
+ go_version: [1.15, 1.16]
+ steps:
+
+ - name: Set up Go 1.x
+ uses: actions/setup-go@v2
+ with:
+ go-version: ${{ matrix.go_version }}
+
+ - name: Check out code into the Go module directory
+ uses: actions/checkout@v2
+
+ - name: Setup examples for testing
+ working-directory: _examples
+ run: ./.github/scripts/setup_examples_test.bash
+
+ - name: Test examples
+ working-directory: _examples
+ run: go test -v -mod=mod -cover -race ./...
diff --git a/.travis.yml b/.travis.yml
index f2234e48..795436ba 100644
--- a/.travis.yml
+++ b/.travis.yml
@@ -30,6 +30,5 @@ script:
after_script:
# examples
- cd ./_examples
- - go get ./...
- - go test -count=1 -v -cover -race ./...
+ - go test -v -mod=mod -cover -race ./...
- cd ../
diff --git a/README.md b/README.md
index 75663ec1..7542541b 100644
--- a/README.md
+++ b/README.md
@@ -222,14 +222,44 @@ Venkatt Guhesan" title="vguhesan" with="75" style="width:75px;max-width:75px;hei
## 📖 Learning Iris
+### Create a new project
+
```sh
$ mkdir myapp
$ cd myapp
$ go mod init myapp
$ go get github.com/kataras/iris/v12@master # or @v12.2.0-alpha2
-$ go mod download
```
+Install on existing project
+
+```sh
+$ cd myapp
+$ go get github.com/kataras/iris/v12@master
+```
+
+
+
+Install with a go.mod file
+
+```txt
+module myapp
+
+go 1.16
+
+require github.com/kataras/iris/v12 master # or v12.2.0-alpha2
+```
+
+**Run**
+
+```sh
+$ go mod download
+$ go run main.go
+# OR go run -mod=mod main.go
+```
+
+
+
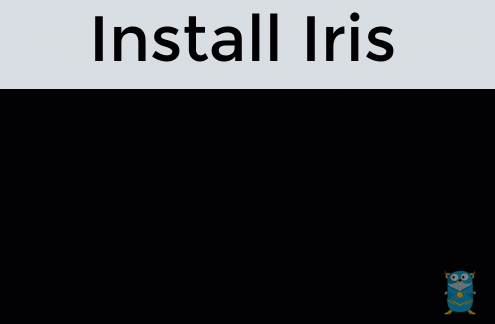
Iris contains extensive and thorough **[documentation](https://www.iris-go.com/docs)** making it easy to get started with the framework.
diff --git a/_examples/README.md b/_examples/README.md
index 638086ab..3f689aef 100644
--- a/_examples/README.md
+++ b/_examples/README.md
@@ -9,7 +9,6 @@
* [MySQL, Groupcache & Docker](database/mysql)
* [MongoDB](database/mongodb)
* [Sqlx](database/orm/sqlx/main.go)
- * [Xorm](database/orm/xorm/main.go)
* [Gorm](database/orm/gorm/main.go)
* [Reform](database/orm/reform/main.go)
* HTTP Server
diff --git a/_examples/auth/basicauth/database/README.md b/_examples/auth/basicauth/database/README.md
index 8cdbe94f..b59433eb 100644
--- a/_examples/auth/basicauth/database/README.md
+++ b/_examples/auth/basicauth/database/README.md
@@ -14,7 +14,7 @@ $ docker-compose up --build
### Install (Manually)
-Run `go build` or `go run main.go` and read below.
+Run `go build -mod=mod` or `go run -mod=mod main.go` and read below.
#### MySQL
diff --git a/_examples/database/mysql/README.md b/_examples/database/mysql/README.md
index b11b92fd..1f218aac 100644
--- a/_examples/database/mysql/README.md
+++ b/_examples/database/mysql/README.md
@@ -76,7 +76,7 @@ $ docker-compose up --build
### Install (Manually)
-Run `go build` or `go run main.go` and read below.
+Run `go build -mod=mod` or `go run -mod=mod main.go` and read below.
#### MySQL
diff --git a/_examples/database/orm/xorm/main.go b/_examples/database/orm/xorm/main.go
deleted file mode 100644
index 8308a5f9..00000000
--- a/_examples/database/orm/xorm/main.go
+++ /dev/null
@@ -1,80 +0,0 @@
-// Package main shows how an orm can be used within your web app
-// it just inserts a column and select the first.
-package main
-
-import (
- "time"
-
- "github.com/kataras/iris/v12"
-
- _ "github.com/mattn/go-sqlite3"
- "xorm.io/xorm"
-)
-
-/*
- go get -u github.com/mattn/go-sqlite3
- go get -u xorm.io/xorm
-
- If you're on win64 and you can't install go-sqlite3:
- 1. Download: https://sourceforge.net/projects/mingw-w64/files/latest/download
- 2. Select "x86_x64" and "posix"
- 3. Add C:\Program Files\mingw-w64\x86_64-7.1.0-posix-seh-rt_v5-rev1\mingw64\bin
- to your PATH env variable.
-
- Docs: https://gitea.com/xorm/xorm
-*/
-
-// User is our user table structure.
-type User struct {
- ID int64 // auto-increment by-default by xorm
- Version string `xorm:"varchar(200)"`
- Salt string
- Username string
- Password string `xorm:"varchar(200)"`
- Languages string `xorm:"varchar(200)"`
- CreatedAt time.Time `xorm:"created"`
- UpdatedAt time.Time `xorm:"updated"`
-}
-
-func main() {
- app := iris.New()
-
- orm, err := xorm.NewEngine("sqlite3", "./test.db")
- if err != nil {
- app.Logger().Fatalf("orm failed to initialized: %v", err)
- }
- iris.RegisterOnInterrupt(func() {
- orm.Close()
- })
-
- if err = orm.Sync2(new(User)); err != nil {
- app.Logger().Fatalf("orm failed to initialized User table: %v", err)
- }
-
- app.Get("/insert", func(ctx iris.Context) {
- user := &User{Username: "kataras", Salt: "hash---", Password: "hashed", CreatedAt: time.Now(), UpdatedAt: time.Now()}
- orm.Insert(user)
-
- ctx.Writef("user inserted: %#v", user)
- })
-
- app.Get("/get", func(ctx iris.Context) {
- user := User{ID: 1}
- found, err := orm.Get(&user) // fetch user with ID.
- if err != nil {
- ctx.StopWithError(iris.StatusInternalServerError, err)
- return
- }
-
- if !found {
- ctx.StopWithText(iris.StatusNotFound, "User with ID: %d not found", user.ID)
- return
- }
-
- ctx.Writef("User Found: %#v", user)
- })
-
- // http://localhost:8080/insert
- // http://localhost:8080/get
- app.Listen(":8080")
-}
diff --git a/_examples/desktop/blink/main.go b/_examples/desktop/blink/main.go
index a771727f..f35979e0 100644
--- a/_examples/desktop/blink/main.go
+++ b/_examples/desktop/blink/main.go
@@ -10,7 +10,7 @@ import (
const addr = "127.0.0.1:8080"
/*
- $ go build -ldflags -H=windowsgui -o myapp.exe
+ $ go build -mod=mod -ldflags -H=windowsgui -o myapp.exe
$ ./myapp.exe # run the app
*/
func main() {
diff --git a/_examples/desktop/lorca/main.go b/_examples/desktop/lorca/main.go
index eb5fe18f..dfd001dc 100644
--- a/_examples/desktop/lorca/main.go
+++ b/_examples/desktop/lorca/main.go
@@ -8,7 +8,7 @@ import (
const addr = "127.0.0.1:8080"
/*
- $ go build -ldflags="-H windowsgui" -o myapp.exe # build for windows
+ $ go build -mod=mod -ldflags="-H windowsgui" -o myapp.exe # build for windows
$ ./myapp.exe # run
*/
func main() {
diff --git a/_examples/desktop/webview/main.go b/_examples/desktop/webview/main.go
index e354a953..9b3abe6d 100644
--- a/_examples/desktop/webview/main.go
+++ b/_examples/desktop/webview/main.go
@@ -13,7 +13,7 @@ const addr = "127.0.0.1:8080"
# http://tdm-gcc.tdragon.net/download
#
#
- $ go build -ldflags="-H windowsgui" -o myapp.exe # build for windows
+ $ go build -mod=mod -ldflags="-H windowsgui" -o myapp.exe # build for windows
$ ./myapp.exe # run
#
#
diff --git a/_examples/sessions/database/redis/main.go b/_examples/sessions/database/redis/main.go
index 034f9769..81a15c12 100644
--- a/_examples/sessions/database/redis/main.go
+++ b/_examples/sessions/database/redis/main.go
@@ -15,8 +15,7 @@ import (
// 1. Install Redis:
// 1.1 Windows: https://github.com/ServiceStack/redis-windows
// 1.2 Other: https://redis.io/download
-// 2. go mod download
-// 3. go run main.go
+// 2. Run command: go run -mod=mod main.go
//
// Tested with redis version 3.0.503.
func main() {
diff --git a/_examples/url-shortener/main.go b/_examples/url-shortener/main.go
index 5e140d6f..ff6bcb4b 100644
--- a/_examples/url-shortener/main.go
+++ b/_examples/url-shortener/main.go
@@ -5,7 +5,7 @@
// $ go get go.etcd.io/bbolt/...
// $ go get github.com/google/uuid
// $ cd $GOPATH/src/github.com/kataras/iris/_examples/url-shortener
-// $ go build
+// $ go build -mod=mod
// $ ./url-shortener
package main
diff --git a/_examples/webassembly/client/hello_go114.go b/_examples/webassembly/client/hello_go116.go
similarity index 75%
rename from _examples/webassembly/client/hello_go114.go
rename to _examples/webassembly/client/hello_go116.go
index 83e0413f..a5d60840 100644
--- a/_examples/webassembly/client/hello_go114.go
+++ b/_examples/webassembly/client/hello_go116.go
@@ -9,7 +9,7 @@ import (
)
func main() {
- // GOARCH=wasm GOOS=js /home/$yourusername/go1.14/bin/go build -o hello.wasm hello_go114.go
+ // GOARCH=wasm GOOS=js /home/$yourusername/go1.16/bin/go build -mod=mod -o hello.wasm hello_go116.go
js.Global().Get("console").Call("log", "Hello Webassembly!")
message := fmt.Sprintf("Hello, the current time is: %s", time.Now().String())
js.Global().Get("document").Call("getElementById", "hello").Set("innerText", message)
diff --git a/_examples/webassembly/main.go b/_examples/webassembly/main.go
index 6d611da1..fdf8c0f6 100644
--- a/_examples/webassembly/main.go
+++ b/_examples/webassembly/main.go
@@ -5,8 +5,8 @@ import (
)
/*
-You need to build the hello.wasm first, download the go1.14 and execute the below command:
-$ cd client && GOARCH=wasm GOOS=js /home/$yourname/go1.14/bin/go build -o hello.wasm hello_go114.go
+You need to build the hello.wasm first, download the go1.16 and execute the below command:
+$ cd client && GOARCH=wasm GOOS=js /home/$yourname/go1.16/bin/go build -mod=mod -o hello.wasm hello_go116.go
*/
func main() {